UPDATE:2024年09月05日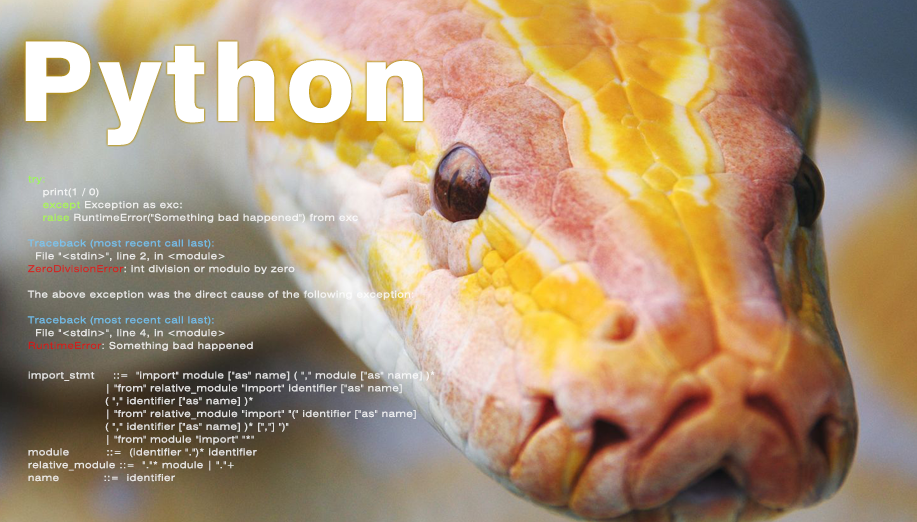
Python 辞書(dict) 連想配列 -入門編-
Pythonリファレンス
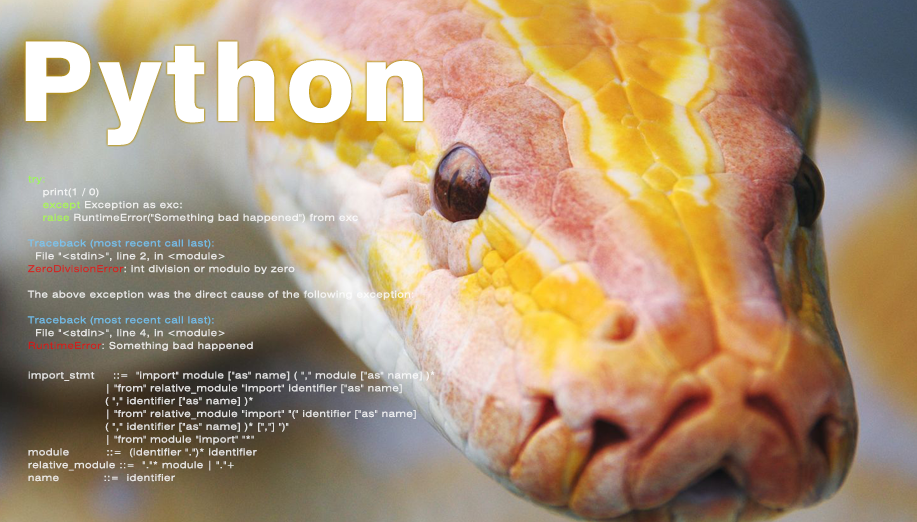
Python3
目次 [閉じる]
Python にも他の言語と同様に複数の要素を同時に扱う機能があります。リスト(list)、タプル(tuple)、辞書(dict)、集合(set)の4種類です。一般的には配列などとも言います。タプル(tuple) や リスト(list) が任意の整数値をインデックスとした要素を順番に管理するシーケンス型であるのに対して、辞書(dict) の場合、一意のkey(キー) と 値(value)を1つのセットとして各要素を構成します。集合(set)の場合、一意のkey(キー)で各要素を構成します。
Python の辞書(dict)
辞書(dict) は、一意の key(キー) と value(値) をペアにして各要素を構成しています。連想配列に近いものです。key(キー) は文字列の場合が多いですが、Python のイミュータブル型であれば扱えます。整数、浮動小数点数、ブール値、float、タプルなどです。辞書は、リスト や タプル のような順序の管理がありません。順番による管理が必要であれば、リスト や タプル を利用するのが良いでしょう。
辞書(dict)の作成と取り出し
「{ } (波括弧)」による作成と取り出し
「{ } (波括弧)」を使用して 辞書 を作成できます。各要素を key:value のペアとして、「, (カンマ)」で区切って並べます。
辞書(dict)の作成
要素数が0個の辞書を作成してみます。
1 2 3 | # -*- coding: utf-8 -*- dictData = {} print(dictData) |
実行結果
{}
空の状態の 辞書 です。
dictData の型を確認すると、
1 2 3 | # -*- coding: utf-8 -*- dictData = {} print(type(dictData)) |
実行結果
<class 'dict'>
dict と表示されます。
要素数が0個以上の 辞書 を作成して取得
key も value も文字列にして複数要素を格納した 辞書 を作成します。格納したい各要素を「,(カンマ)」で区切ります。
1 2 3 4 5 6 7 8 9 10 | # -*- coding: utf-8 -*- #複数要素を格納した辞書の作成 dictData = { 'breakfast':'I did not eat breakfast.', 'lunch':'I ate a hamburger at a fast food store.', 'dinner':'I ate a steak at the restaurant.', } #表示 print(dictData) print(dictData['lunch']) |
実行結果
{'breakfast': 'I did not eat breakfast.', 'lunch': 'I ate a hamburger at a fast food store.', 'dinner': 'I ate a steak at the restaurant.'}
I ate a hamburger at a fast food store.
I ate a hamburger at a fast food store.
for文で取得表示させます。
1 2 3 4 5 | # -*- coding: utf-8 -*- #for文 for key in dictData: print(key) print(dictData[key]) |
実行結果
breakfast
I did not eat breakfast.
lunch
I ate a hamburger at a fast food store.
dinner
I ate a steak at the restaurant.
I did not eat breakfast.
lunch
I ate a hamburger at a fast food store.
dinner
I ate a steak at the restaurant.
key を文字列以外で 辞書 を作成
辞書 の key を文字列以外の型で作成してみます。for文で取得表示させ、key の型も確認してみます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | # -*- coding: utf-8 -*- #keyを文字列以外にした辞書の作成 keyname = 'tuple-name',#タプル dictData = { 100:'int-value', #key整数 3.14:'float-value', #key浮動小数点数 True:'bool-value', #keyブール値 False:'bool-value', #keyブール値 keyname[0]:'tuple-value', #keyタプル } #for文で取得 for key in dictData: print(key) print(typekey)) print(dictData[key]) print('------') |
実行結果
100
<class 'int'>
int-value
——
3.14
<class 'float'>
float-value
——
True
<class 'bool'>
bool-value
——
False
<class 'bool'>
bool-value
——
('tuple-name',)
<class 'tuple'>
tuple-value
——
<class 'int'>
int-value
——
3.14
<class 'float'>
float-value
——
True
<class 'bool'>
bool-value
——
False
<class 'bool'>
bool-value
——
('tuple-name',)
<class 'tuple'>
tuple-value
——
key は基本的に文字列で指定することが多いですが、文字列型以外も利用可能です。
key と value の取得
key と value の取得
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | # -*- coding: utf-8 -*- ### 辞書の作成 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } #items()で取得 listData = list(dictData.items()) print(list(dictData.items())) print(listData[0][0]) #for文で取得 for key, value in dictData.items(): print (key + ':' + value) |
実行結果
[('name', 'Bob'), ('mail', 'bob@mail.com'), ('address', '1-1-1 Nihonbashi Chuo-ku, Tokyo')]
name
name:Bob
mail:bob@mail.com
address:1-1-1 Nihonbashi Chuo-ku, Tokyo
name
name:Bob
mail:bob@mail.com
address:1-1-1 Nihonbashi Chuo-ku, Tokyo
key の取得
keys() によって 辞書 の値を取得できます。取得した キー名 を list() によって リストに格納します。
1 2 3 4 5 6 7 8 9 10 11 12 13 | # -*- coding: utf-8 -*- ### key の取得 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print(dictData.keys()) #listにして取得 dictKeys = list(dictData.keys()) print(dictKeys[0]) print(dictKeys[1]) print(dictKeys[2]) |
実行結果
dict_keys(['name', 'mail', 'address'])
name
mail
address
name
address
value の取得
values() によって 辞書 の値を取得できます。
1 2 3 4 5 6 7 8 9 10 11 12 13 | # -*- coding: utf-8 -*- ### value の取得 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print(dictData.values()) #listにして取得 dictValues = list(dictData.values()) print(dictValues[0]) print(dictValues[1]) print(dictValues[2]) |
実行結果
dict_values(['Bob', 'bob@mail.com', '1-1-1 Nihonbashi Chuo-ku, Tokyo'])
Bob
bob@mail.com
1-1-1 Nihonbashi Chuo-ku, Tokyo
Bob
bob@mail.com
1-1-1 Nihonbashi Chuo-ku, Tokyo
key による取得
キー名 を指定して値を取得できます。
1 2 3 4 5 6 7 8 9 10 | # -*- coding: utf-8 -*- ### key による取得 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print(dictData['name']) print(dictData['mail']) print(dictData['address']) |
実行結果
Bob
bob@mail.com
1-1-1 Nihonbashi Chuo-ku, Tokyo
bob@mail.com
1-1-1 Nihonbashi Chuo-ku, Tokyo
要素の追加
キー名 を指定して要素を追加できます。既にその キー名 がある場合は要素の値が更新されます。
1 2 3 4 5 6 7 8 9 | # -*- coding: utf-8 -*- ### keyによる要素の追加 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } dictData['tel'] = '000-1111-2222' print(dictData) |
実行結果
{'name': 'Bob', 'mail': 'bob@mail.com', 'address': '1-1-1 Nihonbashi Chuo-ku, Tokyo', 'tel': '000-1111-2222'}
要素の削除
key名 を指定して delキーワード により要素を削除できます。
1 2 3 4 5 6 7 8 9 10 | # -*- coding: utf-8 -*- ### keyによる要素の削除 delキーワード dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print(dictData) del dictData['name'] print(dictData) |
実行結果
{'name': 'Bob', 'mail': 'bob@mail.com', 'address': '1-1-1 Nihonbashi Chuo-ku, Tokyo'}
{'mail': 'bob@mail.com', 'address': '1-1-1 Nihonbashi Chuo-ku, Tokyo'}
{'mail': 'bob@mail.com', 'address': '1-1-1 Nihonbashi Chuo-ku, Tokyo'}
要素の変更
key名 を指定して要素の値を変更できます。
1 2 3 4 5 6 7 8 9 10 | # -*- coding: utf-8 -*- ### keyによる要素の変更 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print(dictData['name']) dictData['name'] = 'Tom' print(dictData['name']) |
実行結果
Bob
Tom
Tom
辞書の結合
辞書 と 辞書 を統合する場合、 update()関数 を使用します。ある辞書のキーと値を別の辞書にコピーすることが出来ます。
1 2 3 4 5 6 7 8 9 10 11 12 13 | # -*- coding: utf-8 -*- ### updateによる辞書の結合 dictData1 = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } dictData2 = { 'tel':'000-1111-2222', 'gender':'male' } dictData1.update(dictData2) print(dictData1) |
実行結果
{'name': 'Bob', 'mail': 'bob@mail.com', 'address': '1-1-1 Nihonbashi Chuo-ku, Tokyo', 'tel': '000-1111-2222', 'gender': 'male'}
clear() による要素の一括削除
clear() を使用すると 辞書 の キー(key) と 値(value) をすべて削除することが出来ます。
1 2 3 4 5 6 7 8 9 | # -*- coding: utf-8 -*- ### clear()によるすべての要素の一括削除 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } dictData.clear() print(dictData) |
実行結果
{}
辞書 に {} を代入しても空にすることが出来ます。
1 2 3 4 5 6 7 8 9 | # -*- coding: utf-8 -*- ### clear()によるすべての要素の一括削除 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } dictData = {} print(dictData) |
{}
in による要素の確認
辞書 が特定の key名 を保持しているかどうか調べるには in を使用します。key名 が存在する場合は 論理値 の True を返し、存在しない場合は False を返します。
1 2 3 4 5 6 7 8 9 | # -*- coding: utf-8 -*- ### in によるkeyの確認 dictData = { 'name':'Bob', 'mail':'bob@mail.com', 'address':'1-1-1 Nihonbashi Chuo-ku, Tokyo', } print('name' in dictData) print('tel' in dictData) |
実行結果
True
False
False
タグ(=記事関連ワード)
日付
投稿日:2017年9月19日
最終更新日:2024年09月05日
最終更新日:2024年09月05日
このカテゴリの他のページ
この記事へのコメント
トラックバックurl
https://wepicks.net/python-reference-dict/trackback/